Data Science: Deep Learning and Neural Networks in Python
- Description
- Curriculum
- FAQ
- Reviews
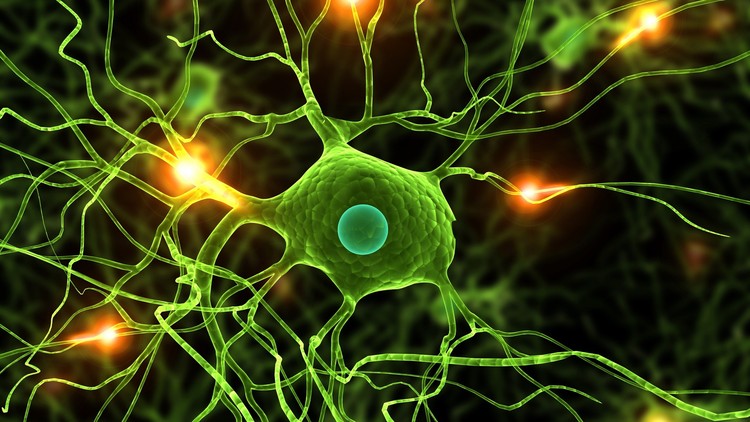
Deep Learning and Neural Networks in Python
This course will get you started in building your FIRST artificial neural network using deep learning techniques. Following my previous course on logistic regression, we take this basic building block, and build full-on non-linear neural networks right out of the gate using Python and Numpy. All the materials for this course are FREE.
“If you can’t implement it, you don’t understand it”
- Or as the great physicist Richard Feynman said: “What I cannot create, I do not understand”.
- My courses are the ONLY courses where you will learn how to implement machine learning algorithms from scratch
- Other courses will teach you how to plug in your data into a library, but do you really need help with 3 lines of code?
- After doing the same thing with 10 datasets, you realize you didn’t learn 10 things. You learned 1 thing, and just repeated the same 3 lines of code 10 times…
Deep Learning and Neural Networks in Python:
- calculus (taking derivatives)
- matrix arithmetic
- probability
- Python coding: if/else, loops, lists, dicts, sets
- Numpy coding: matrix and vector operations, loading a CSV file
- Be familiar with basic linear models such as linear regression and logistic regression
WHATÂ ORDERÂ SHOULDÂ IÂ TAKEÂ YOURÂ COURSESÂ IN?:
- Check out the lecture “Machine Learning and AIÂ Prerequisite Roadmap” (available in the FAQ of any of my courses, including the free Numpy course)
-
12Prediction: Section Introduction and Outline
-
13From Logistic Regression to Neural Networks
-
14Interpreting the Weights of a Neural Network
-
15Softmax
What's the function we use to classify more than 2 things?
-
16Sigmoid vs. Softmax
-
17Feedforward in Slow-Mo (part 1)
-
18Feedforward in Slow-Mo (part 2)
-
19Where to get the code for this course
-
20Softmax in Code
How do we code the softmax in Python?
-
21Building an entire feedforward neural network in Python
Let's extend softmax and code the entire calculation from input to output.
-
22E-Commerce Course Project: Pre-Processing the Data
-
23E-Commerce Course Project: Making Predictions
-
24Prediction Quizzes
-
25Prediction: Section Summary
-
26Suggestion Box
-
27Training: Section Introduction and Outline
-
28What do all these symbols and letters mean?
-
29What does it mean to "train" a neural network?
-
30How to Brace Yourself to Learn Backpropagation
-
31Categorical Cross-Entropy Loss Function
-
32Training Logistic Regression with Softmax (part 1)
-
33Training Logistic Regression with Softmax (part 2)
-
34Backpropagation (part 1)
-
35Backpropagation (part 2)
-
36Backpropagation in code
How to code bacpropagation in Python using numpy operations vs. slow for loops.
-
37Backpropagation (part 3)
-
38The WRONG Way to Learn Backpropagation
-
39E-Commerce Course Project: Training Logistic Regression with Softmax
-
40E-Commerce Course Project: Training a Neural Network
-
41Training Quiz
-
42Training: Section Summary
-
43Practical Issues: Section Introduction and Outline
-
44Donut and XOR Review
What are the donut and XOR problems again?
-
45Donut and XOR Revisited
We look again at the XOR and donut problem from logistic regression. The features are now learned automatically.
-
46Neural Networks for Regression
-
47Common nonlinearities and their derivatives
sigmoid, tanh, relu along with their derivatives
-
48Practical Considerations for Choosing Activation Functions
-
49Hyperparameters and Cross-Validation
Tips on choosing learning rate, regularization penalty, number of hidden units, and number of hidden layers.
-
50Manually Choosing Learning Rate and Regularization Penalty
-
51Why Divide by Square Root of D?
-
52Practical Issues: Section Summary
-
53TensorFlow plug-and-play example
A look at Google's new TensorFlow library.
-
54Visualizing what a neural network has learned using TensorFlow Playground
-
55Where to go from hereWhat did you learn? What didn't you learn? Where can you learn more?
-
56You know more than you think you know
-
57How to get good at deep learning + exercises
-
58Deep neural networks in just 3 lines of code with Sci-Kit Learn
-
59Facial Expression Recognition Project Introduction
-
60Facial Expression Recognition Problem Description
-
61The class imbalance problem
-
62Utilities walkthrough
-
63Facial Expression Recognition in Code (Binary / Sigmoid)
-
64Facial Expression Recognition in Code (Logistic Regression Softmax)
-
65Facial Expression Recognition in Code (ANN Softmax)
-
66Facial Expression Recognition Project Summary