Java Fundamentals: Learn the Basics of Java
- Description
- Curriculum
- FAQ
- Reviews
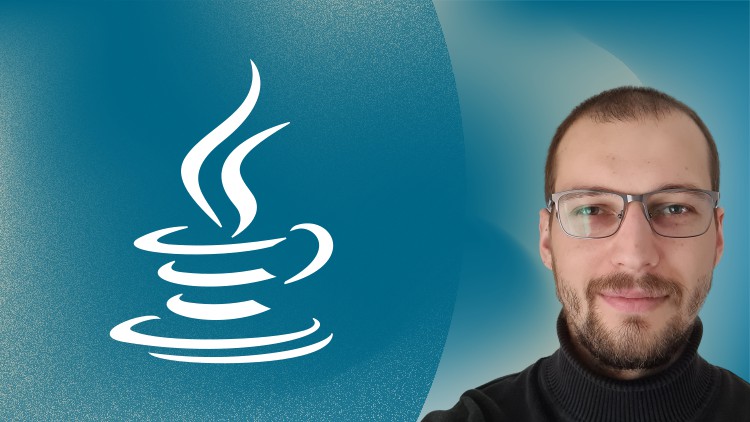
Java fundamentals Learn Java basics:
Java has been around for a long time and has been the driving force in the IT industry. Additionally, it’s one of the most popular and sustainable programming languages ever created. Its flexibility is unmatched as it’s used in virtually all domains of software development. Furthermore, Java is often used for building web applications, desktop applications, mobile applications, game engines, etc.
This course will help you build strong foundations by mastering the fundamentals of the Java programming language. Moreover, we will go over all the essentials and leave no stone unturned, in your path to becoming a successful Java developer.
Whether you’re starting from scratch or looking to reinforce existing knowledge, this comprehensive curriculum will ensure you build a strong foundation. After this course, you will be ready to pursue your career path as a Java developer.
You will have plenty of options in front of you. Additionally, whether you choose to build web applications, desktop applications, mobile applications, or all of them, as long as you have strong knowledge of the fundamentals, switching between different technology stacks is easy.
I’ve structured this course in such a way that lessons aren’t too long and get straight to the point. Furthermore, at the end of each section, there is a quick recap, quizzes, and coding exercises. None of the exercises are mandatory, but I encourage you to try them at least once.
There is already plenty of free learning material out there. However, it’s easy to get lost and stuck, not knowing where to go next. Java fundamentals learn Java basics course is well-structured and covers the whats, whys, and hows, all in one place
-
1Introduction
-
2What is Java?
-
3Installing Java (Windows, Mac)
Windows and Mac
You can download the Java Development Kit (JDK) at: https://www.oracle.com/java/technologies/downloads/
I recommend downloading the latest suggested version.
-
4Installing Java (Linux)
Ubuntu
To install the JDK on Ubuntu, you can use apt. Here is a good guide by Linode:
https://www.linode.com/docs/guides/how-to-install-openjdk-ubuntu-22-04/#how-to-install-openjdkIf you want to use multiple versions of Java, follow this guide:
https://aboullaite.me/switching-between-java-versions-on-ubuntu-linuxFedora
On Fedora you can use dnf to install OpenJDK:
https://docs.fedoraproject.org/en-US/quick-docs/installing-javaOpenSUSE
On OpenSUSE the installation is more complicated due to Oracle's licensing issues. However, it's not impossible and OpenSUSE provides a guide on how to install Java:
https://en.opensuse.org/SDB:Installing_JavaArch Linux
Arch Linux has a utility for easily installing Java:
https://wiki.archlinux.org/title/javaRHEL
On Red Hat Linux you can install Java with yum:
https://access.redhat.com/documentation/en-us/red_hat_build_of_openjdk/21/html/installing_and_using_red_hat_build_of_openjdk_21_on_rhel/installing-openjdk11-on-rhel8_openjdkGentoo
To install Java on Gentoo, you can follow these instructions from their wiki page:
https://wiki.gentoo.org/wiki/Java -
5Installing IntelliJ (Windows, Mac)
-
6Installing IntelliJ (Linux)
Flatpak
Official Flatpak image: https://flathub.org/apps/com.jetbrains.IntelliJ-IDEA-Community
flatpak install flathub com.jetbrains.IntelliJ-IDEA-Community
Snap
Official Snap image:
sudo snap install intellij-idea-community --classic
https://snapcraft.io/intellij-idea-community
AppImage
Not available at the time of me writing this.
Manual Install
Download .tar.gz file from the IntelliJ IDEA website, unpack it, and you will find instructions setting everything up. https://www.jetbrains.com/idea/download/?section=linux
-
7Our First Java Project
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/01_getting_started/03_our_first_java_project.md
-
8Java Architecture Overview
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/01_getting_started/04_java_architecture_overview.md
-
9Quick Recap
-
10How to Take This Course
-
11Source Code
The source code (and more) can be found in my public GitHub repository:
https://github.com/bezbos/complete-java-course
-
12Where to Find Me
You can find me on the following platforms (no links due to Udemy rules, so I've placed my username or name):
YouTube: bezbos
LinkedIn: Boško Bezik
Medium: bezbos.
GitHub: bezbos
StackOverflow: bezbos
-
13Getting Started Quiz
-
14Variables
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/01_variables.md
-
15Final Variables
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/02_final_variables.md
-
16Expressions & Statements
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/03_expressions_statements_blocks.md
-
17Comments
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/04_comments.md
-
18Primitive Types
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/05_primitive_types.md
-
19Floating Point Primitives
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/06_floating_point_and_big_decimal.md
-
20Strings
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/07_strings.md
-
21String Escape Sequences
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/08_string_escape_sequences.md
-
22String Formatting
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/09_formatting_strings.md
-
23Multiline Strings (Text Blocks)
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/10_multiline_strings_text_blocks.md
-
24Primitive Type Casting
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/11_primitive_type_casting.md
-
25Converting Strings and Numbers
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/12_conversion_between_strings_and_numbers.md
-
26Static Typing and `var` Type Name
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/13_var_typename.md
-
27Compile-time vs Runtime
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/16_compile_time_vs_runtime.md
-
28Default Values
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/14_default_values.md
-
29Literals and Literal Formatting
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/02_data_types_and_variables/15_literals_and_literal_formatting.md
-
30Quick Recap
-
31Data Types and Variables Quiz
-
32Addition with Variable Values
-
33String Concatenation
-
34String Manipulation
-
35String Formatting
-
36String Escape Sequences
-
37Replacing Strings
-
38Arithmetic Operators
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/03_operators/01_arithmetic_operators.md
-
39Advanced Arithmetic Operations
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/03_operators/02_advanced_arithmetic_operations.md
-
40Logical Operators
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/03_operators/03_logical_operators.md
-
41Operator Precedence
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/03_operators/04_operator_precedence.md
-
42Bitwise and Bitshift Operators
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/03_operators/05_bitwise_and_bitshift_operators.md
-
43Quick Recap
-
44Operators Quiz
-
45Arrays
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/04_arrays/01_arrays.md
-
46Multidimensional Arrays
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/04_arrays/02_multidimensional_arrays.md
-
47Array Methods
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/04_arrays/03_array_methods.md
-
48Quick Recap
-
49Arrays Quiz
-
50`if` Statement
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/05_conditional_statements/01_if_statement.md
-
51Reading Console Input
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/05_conditional_statements/02_reading_console_input.md
-
52`switch` Statement
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/05_conditional_statements/03_switch_statement.md
-
53`switch` Expression
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/05_conditional_statements/04_switch_expressions.md
-
54Quick Recap
-
55Conditional Statements Quiz
-
56Temperature Checker
-
57Temperature and Weather Checker
-
58Day Teller
-
59`while` Loop
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/01_while_loop.md
-
60`do-while` Loop
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/02_do_while_loop.md
-
61`for` Loop
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/03_for_loop.md
-
62`break` Statement
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/04_break_statement.md
-
63`continue` Statement
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/05_continue_statement.md
-
64Processing Arrays with Loops
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/06_processing_arrays_with_loops.md
-
65`for-each` Loop
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/06_loops/07_enhanced_for_loop.md
-
66Quick Recap
-
67Even Numbers
-
68Sum of Elements
-
69Guess the Number
-
70Extracting Methods
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/01_extracting_methods.md
-
71Effective Naming
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/02_effective_naming.md
-
72Refactoring
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/03_refactoring.md
-
73Avoiding Code Duplication
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/04_avoiding_code_duplication.md
-
74Magic Strings and Numbers
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/05_magic_strings_and_numbers.md
-
75Effective Commenting
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/06_effective_commenting.md
-
76Code Style and Formatting
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/07_code_formatting_and_style.md
-
77Version Control and Collaboration
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/08_version_control_and_collaboration.md
-
78Effective Documentation
Source code:
https://github.com/bezbos/complete-java-course/blob/main/01_java_fundamentals/07_effective_coding/09_effective_documentation.md
-
79Quick Recap
-
80Effective Coding Quiz