The Complete Lua Programming Course: From Zero to Expert!
- Description
- Curriculum
- FAQ
- Reviews
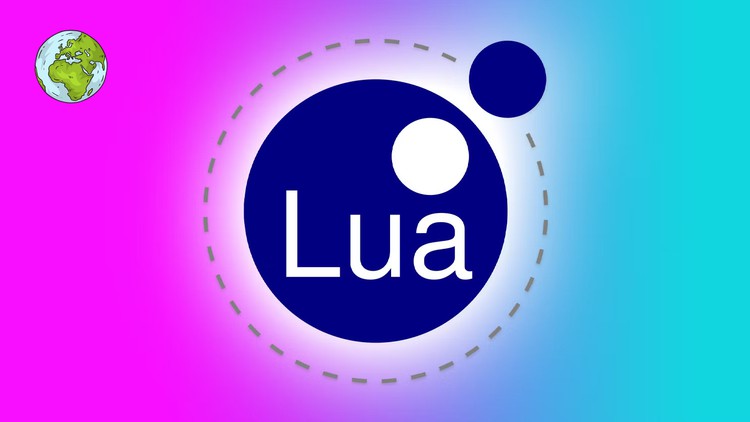
Complete Lua programming course
**Description:**
Embark on a journey to master Lua programming from scratch with our comprehensive course designed for beginners and experienced developers alike. Lua is a powerful scripting language known for its simplicity, flexibility, and versatility, making it an ideal choice for game development, embedded systems, scripting, and more. In this course, you’ll start from the basics and progress to advanced topics, gaining the skills and confidence to become a Lua programming expert.
Through a combination of theory and hands-on projects, you’ll learn the fundamentals of Lua syntax, data types, control structures, functions, and modules. You’ll explore practical examples and real-world scenarios, delving into Lua’s unique features such as coroutines, metatables, and closures. Additionally, you’ll discover best practices for writing clean, efficient, and maintainable Lua code that meets industry standards.
**Requirements:**
– No prior programming experience required.
– Access to a computer with Lua installed (free to download).
– Enthusiasm and dedication to learn Lua programming from scratch.
**Who This Course Is For:**
– Beginners interested in learning Lua programming language from scratch.
– Game developers looking to enhance their skills in Lua scripting for game development.
– Software engineers seeking to add Lua to their programming repertoire for embedded systems or scripting tasks.
– Anyone passionate about programming and eager to become proficient in Lua for various applications.
By the end of this course, you’ll have the knowledge and expertise to write Lua code confidently and tackle complex programming challenges with ease. Whether you’re developing games, scripting automation tasks, or building embedded systems, this Complete Lua Programming Course will equip you with the skills to excel as a Lua programmer from zero to expert.
Enroll now and start your journey to mastering Lua programming today!
Compare Prices from Udemy
-
1Introduction to Lua
On this lecture we make a brief introduction to Lua programming language.
-
2Installing Lua on Windows
On this lecture we learn how to install Lua on Windows.
-
3Installing Lua on Linux
On this lecture we learn how to install Lua on Linux.
-
4Installing Lua on MacOS
On this lecture we learn how to install Lua on MacOS.
-
5Interlude - Factorial Computation
On this interlude we implement a function to compute the factorial of a number.
-
6Lexical Conventions
On this lecture we study the Lexical Conventions in Lua.
-
7Types and Values
On this lecture we introduce the types in Lua.
-
8Booleans and Logical Operators
On this lecture we study booleans and logical operators in Lua.
-
9Input and Output
On this lecture we see the input and output system in Lua.
-
17Problem 1 - Square Roots and Squares
In this lesson we solve the problem 1 of the problems section on Functions.
-
18Problem 2 - Special Prime Number
In this lesson we solve the problem 2 of the problems section on Functions.
-
19Problem 3 - Stamps
In this lesson we solve the problem 3 of the problems section on Functions.
-
20Problem 4 - Related Sum
In this lesson we solve the problem 4 of the problems section on Functions.
-
21Problem 5 - Polynomial Evaluation
In this lesson we solve the problem 5 of the problems section on Functions.
-
24Numerical For
On this lecture we study the numerical for loop in Lua.
We explain the fundamentals of this control structure and practise with some examples.
-
25Generic For and Iterators
On this lecture we study the generic for loop and learn to define iterator functions.
-
26While Loop
On this lecture we introduce the while loop in Lua.
-
27Repeat Statement
On this lecture we study the repeat statement in Lua and practise with some examples.
-
28Problem 1 - Multiplication Table
In this lesson we solve the problem 1 of the problems section on Loops.
-
29Problem 2 - Sum of Squares
In this lesson we solve the problem 2 of the problems section on Loops.
-
30Problem 3 - Number of Digits
In this lesson we solve the problem 3 of the problems section on Loops.
-
31Problem 4 - Reversed Number
In this lesson we solve the problem 4 of the problems section on Loops.
-
32Problem 5 - Cool Numbers
In this lesson we solve the problem 5 of the problems section on Loops.
-
39Introduction to the Section
On this lecture we introduce the stack problems section.
-
40Problem 1 - Palindromic Sequence
In this lesson we solve the problem 1 of the problems section on Stacks.
-
41Important Considerations
On this lecture we solve the problem 1 using the stack data structure.
-
42Problem 2 - Parenthesization Evaluation
On this lesson we solve problem 2 of the problems section on Stacks.
-
43Problem 3 - Smaller on the Left
On this lesson we solve problem 3 of the problems section on Stacks.
-
61Problem 1 - Size
On this lesson we solve problem 1 of the problems section on Binary Trees.
-
62Problem 2 - Height
On this lesson we solve problem 2 of the problems section on Binary Trees.
-
63Problem 3 - Equivalent Trees
On this lesson we solve problem 3 of the problems section on Binary Trees.
-
64Problem 4 - Isomorphism
On this lesson we solve problem 4 of the problems section on Binary Trees.
-
65Problem 5 - Preorder Traversal
We solve problem 5 of the problems section on binary trees.
-
66Problem 6 - Postorder Traversal
We solve problem 6 of the problems section on binary trees.
-
67Problem 7 - Inorder Traversal
We solve problem 7 of the problems section on binary trees.
-
68Problem 8 - Minimum Value
We solve problem 8 of the problems section on binary trees.
-
69Problem 9 - Root to Leaf Paths
We solve problem 9 of the problems section on binary trees.
-
70Introduction to Graphs
On this lesson we introduce Graphs.
-
71Representation of a Graph
On this lesson we study the two main representations of graphs.
The adjacency list and the adjacency matrix.
-
72Implementation of Graphs
On this lecture we learn how to implement the graph data structure in Lua.
-
74Problem 1 - Treasures in a Map
On this lesson we solve problem 1 of the problems section on Depth First Search.
-
75Problem 2 - Number of Rewards
On this lesson we solve problem 2 of the problems section on Depth First Search.
-
76Problem 3 - Forest
On this lesson we solve problem 3 of the problems section on Depth First Search.
-
77Problem 4 - Two Colors
On this lesson we solve problem 4 of the problems section on Depth First Search.